In the world of web application development, efficiency is key. Laravel, a powerful PHP framework, provides an Eloquent ORM (Object-Relational Mapping) for seamless interaction with databases. Eloquent’s join clauses are incredibly potent tools for developers seeking optimal performance and streamlined query logic. Understanding how to effectively implement these joins can significantly improve data retrieval processes in your Laravel projects. Below, we delve into how to use Eloquent joins to their utmost potential.
Understanding Eloquent Joins in Laravel and Their Impact on Query Optimization
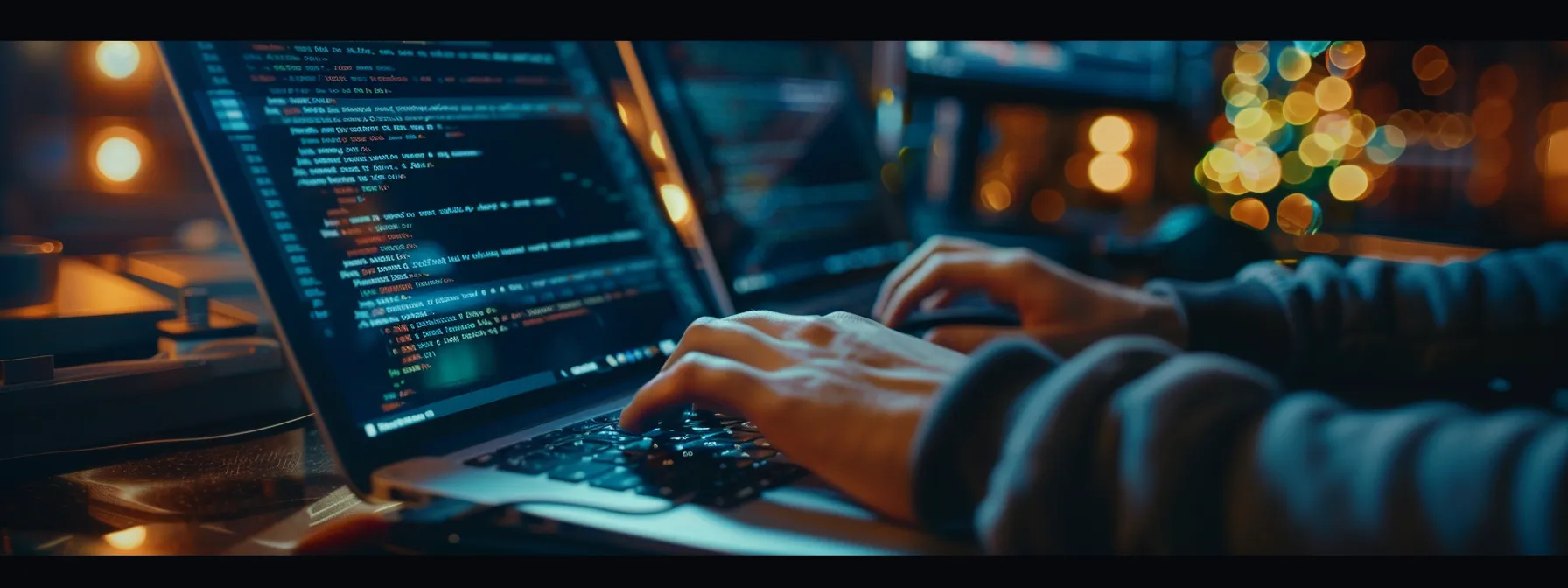
Query optimization in Laravel begins with a solid grasp of Eloquent joins. These methods enable the developer to pull related data from multiple tables effectively. By specifying conditions upon which tables should be joined, Eloquent handles the SQL intricacies, providing a straightforward syntax that hides the complexity of underlying join operations. This simplification can lead to more readable code and less room for errors.
Eloquent’s join capabilities support not just simple foreign key associations, but also more complex conditions and multiple joins within the same query. It’s this versatility that allows Eloquent to accommodate a wide array of database querying requirements. Leveraging these joins correctly can reduce the number of queries made to the database, thereby improving application performance.
Any experienced developer knows that unoptimized queries can become bottlenecks. Eloquent join operations help mitigate this by allowing aggregate functions, where clauses, and other SQL conditions to be applied directly to the joined tables. This means that data can be filtered and sorted before it is even retrieved, saving precious server resources.
Through the use of Laravel eloquent join, developers can achieve more granular control over their queries. Taking full advantage of this feature requires a clear understanding of the database schema and how tables relate to each other.
Implementing Inner Join in Eloquent for Efficient Data Retrieval
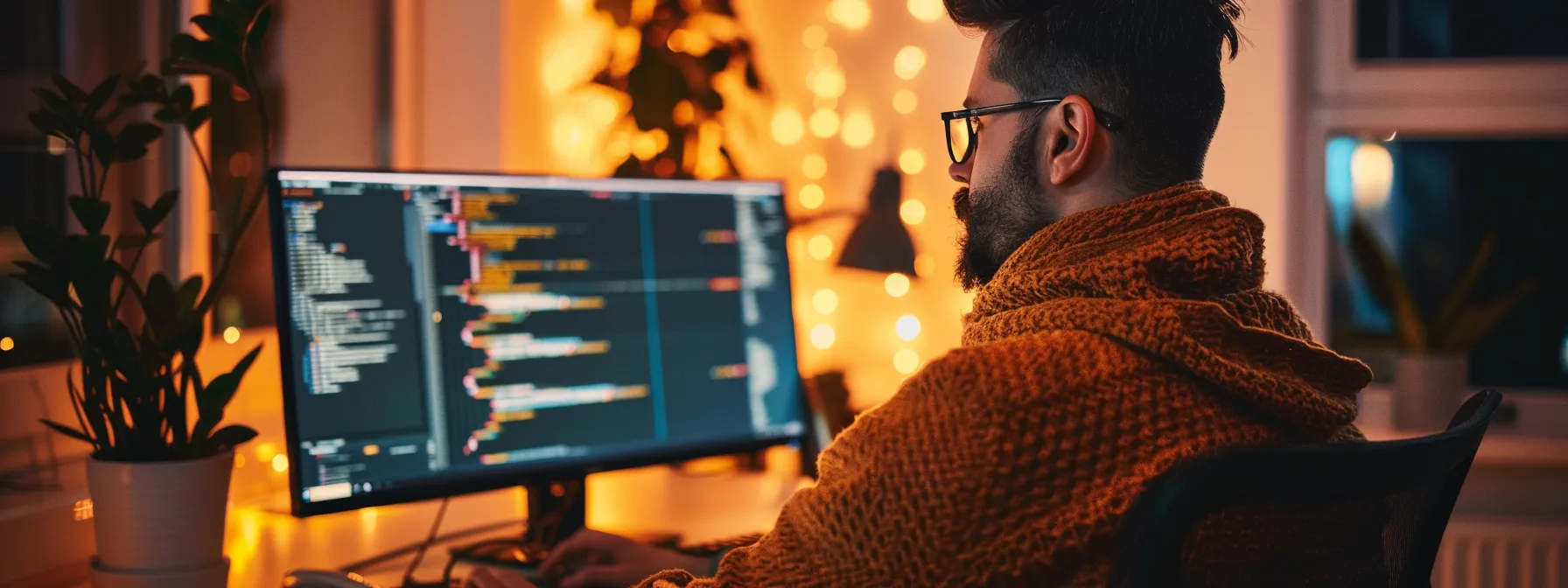
One of the most commonly used join operations in database queries is the inner join. In Laravel’s Eloquent, an inner join can be implemented effortlessly to retrieve records that have matching values in both tables. This selective retrieval is central to preventing superfluous data from being loaded into application memory, thereby optimizing performance.
Eloquent’s inner join clause is not only efficient but also intuitive. Developers can chain these joins directly onto their model queries, maintaining a clean and modular approach to query building. This chaining can significantly reduce the amount of code written and the potential for mistakes, leading to a more maintainable codebase.
Incorporating conditions into your inner joins is another way to fine-tune data retrieval in Laravel. Eloquent supports adding where clauses and other SQL conditionals directly to joins, enabling you to craft precise queries that fetch exactly the data you need.
Exploring Outer Joins With Eloquent: Enhancing Conditional Relationships
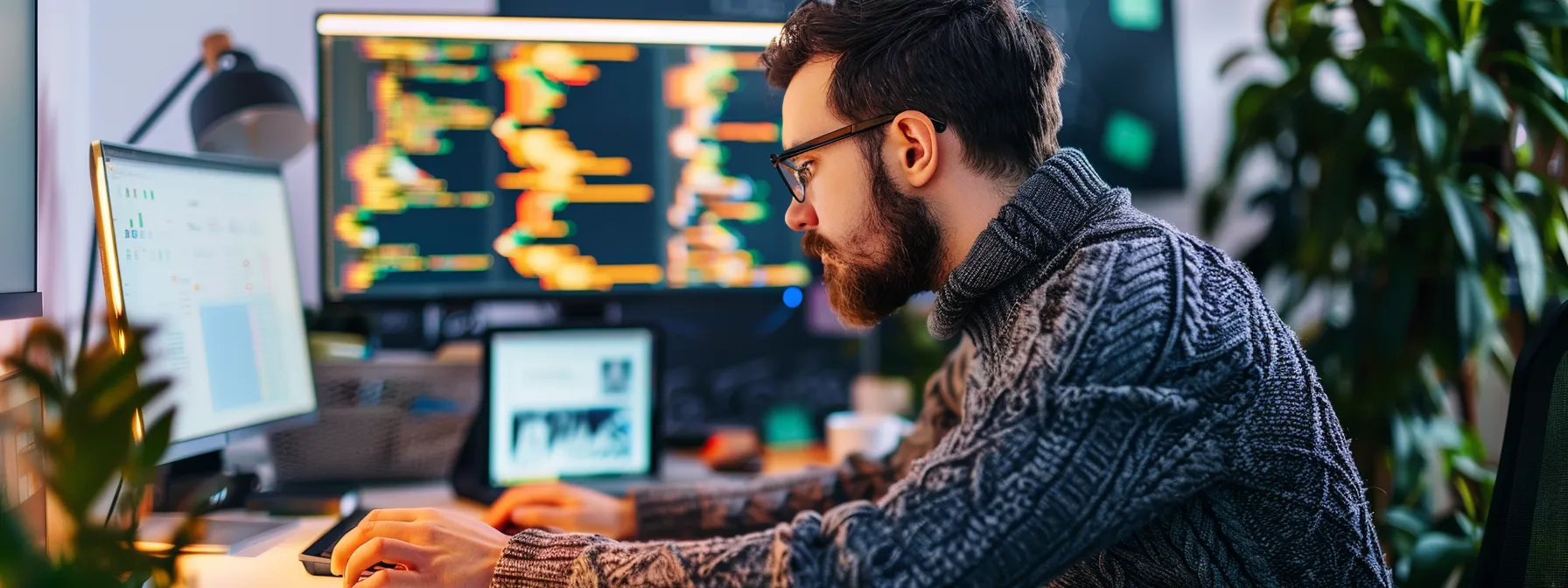
While inner joins fetch records with matching pairs, outer joins are designed to include rows that might not have a corresponding match in the joined table. When working with Laravel’s Eloquent, developers can specify whether they require a left or right outer join, each serving distinct purposes. The inclusion of these non-matching rows can provide a more complete dataset for certain applications.
Left outer joins, in particular, are useful when you want to retrieve all records from the primary table, plus the matching records from the joined table, if available. When applying this in Eloquent, it is necessary to consider the potential for null values in the returned results and handle them accordingly in your application logic.
Right outer joins, less common but equally useful, operate in the reverse. They aim to return all records from the joined table and the matching records from the primary table. In practice, using right outer joins in the Eloquent model has similar considerations to left joins, with careful mapping of null values as one of the pivotal concerns.
Eloquent’s Polymorphic Joins: Simplifying Complex Data Relationships
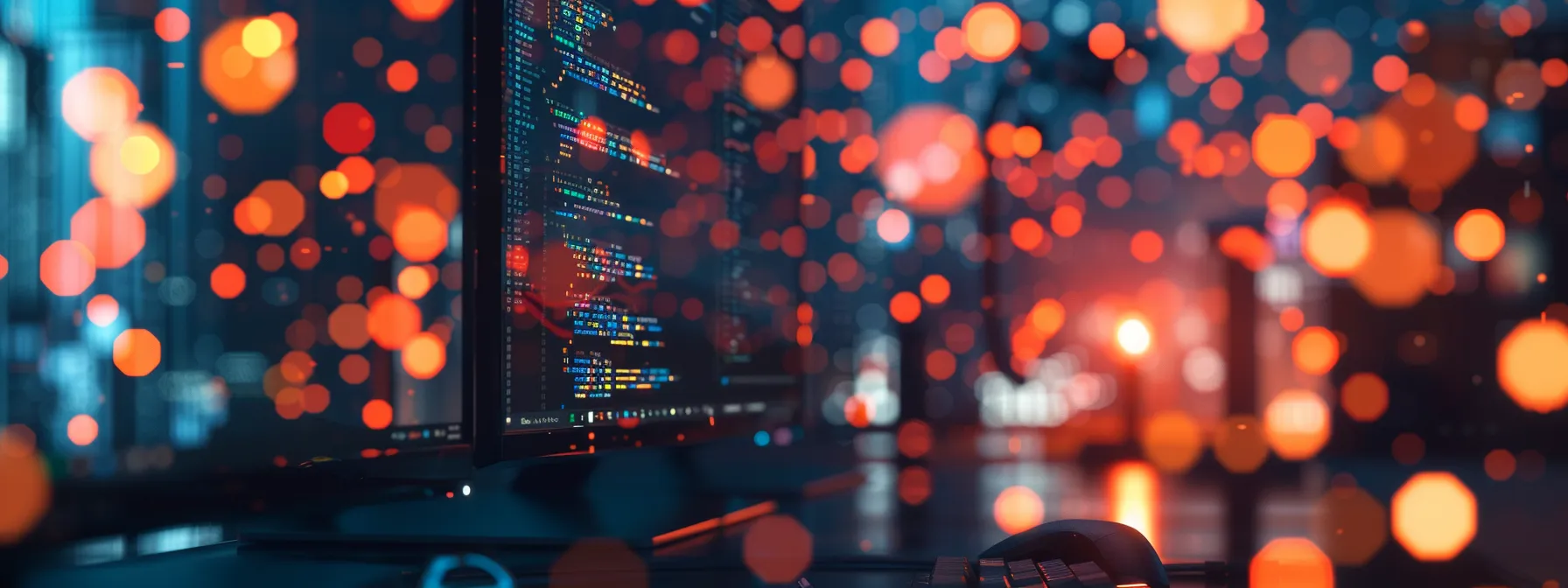
Not all data relationships are straightforward; some have a polymorphic nature, meaning that they can belong to more than one model. Eloquent embraces these complexities with polymorphic joins, making it possible to associate records with multiple models without repetitive foreign keys. This approach streamlines database structures and adds a layer of flexibility to data associations.
When introducing polymorphic joins in Laravel, developers can utilize morphed models that abstract the complexities of these relationships. This abstraction enables a single association method that can dynamically adapt to the related model in context. The result is a cleaner, less convoluted database schema and more readable code within Laravel’s Eloquent ORM.
Utilizing polymorphic joins can also benefit developers by reducing the need for extensive table alterations as new types of relationships are introduced to the application.
Overall, the proper use of Eloquent joins can lead to significant enhancements in Laravel applications, from performance boosts to clearer and more maintainable code.